Adam Dudczak, Marcin Grzejszczak, Jakub Kubryński, Karol Lassak, Olga Maciaszek-Sharma, Mariusz Smykuła
Introduction
Just to make long story short - Accurest is a tool that enables Consumer Driven Contract (CDC) development of JVM-based applications. It is shipped with Contract Definition Language (DSL). Contract definitions are used by Accurest to produce following resources:
-
JSON stub definitions to be used by Wiremock when doing integration testing on the client code (client tests). Test code must still be written by hand, test data is produced by Accurest.
-
Messaging routes if you’re using one. We’re integrating with Spring Integration, Spring Cloud Stream and Apache Camel. You can however set your own integrations if you want to
-
Acceptance tests (in JUnit or Spock) used to verify if server-side implementation of the API is compliant with the contract (server tests). Full test is generated by Accurest.
Accurest moves TDD to the level of software architecture.
Why?
Let us assume that we have a system comprising of multiple microservices:
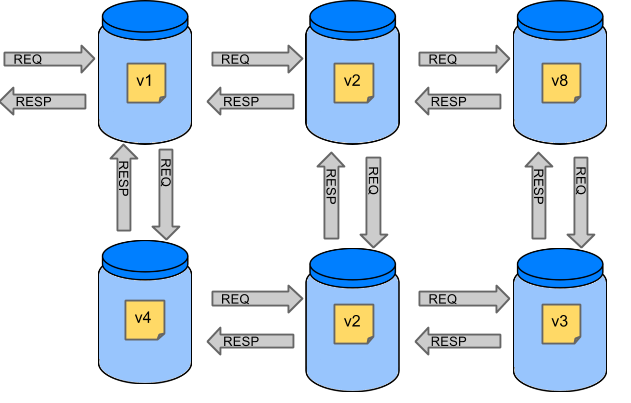
Testing issues
If we wanted to test the application in top left corner if it can communicate with other services then we could do one of two things:
-
deploy all microservices and perform end to end tests
-
mock other microservices in unit / integration tests
Both have their advantages but also a lot of disadvantages. Let’s focus on the latter.
Deploy all microservices and perform end to end tests
Advantages:
-
simulates production
-
tests real communication between services
Disadvantages:
-
to test one microservice we would have to deploy 6 microservices, a couple of databases etc.
-
the environment where the tests would be conducted would be locked for a single suite of tests (i.e. nobody else would be able to run the tests in the meantime).
-
long to run
-
very late feedback
-
extremely hard to debug
Mock other microservices in unit / integration tests
Advantages:
-
very fast feedback
-
no infrastructure requirements
Disadvantages:
-
the implementor of the service creates stubs thus they might have nothing to do with the reality
-
you can go to production with passing tests and failing production
To solve the aforementioned issues Accurest with Stub Runner were created. Their main idea is to give you very fast feedback, without the need to set up the whole world of microservices.
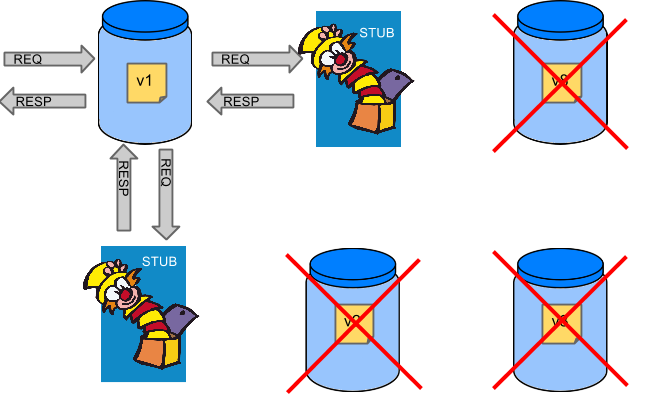
If you work on stubs then the only applications you need are those that your application is using directly.
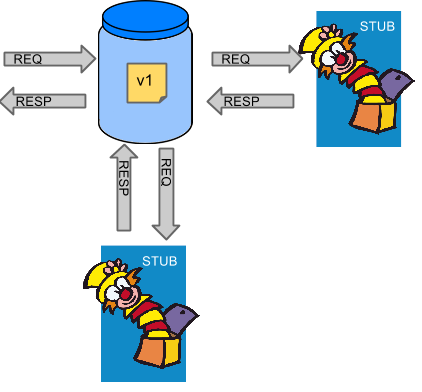
Accurest gives you the certainty that the stubs that you’re using were created by the service that you’re calling. Also if you can use them it means that they were tested against the producer’s side. In other words - you can trust those stubs.
Purposes
The main purposes of Accurest with Stub Runner are:
-
to ensure that WireMock / Messaging stubs (used when developing the client) are doing exactly what actual server-side implementation will do,
-
to promote ATDD method and Microservices architectural style,
-
to provide a way to publish changes in contracts that are immediately visible on both sides,
-
to generate boilerplate test code used on the server side.
Client Side
During the tests you want to have a Wiremock instance / Messaging route up and running that simulates the service Y. You would like to feed that instance with a proper stub definition. That stub definition would need to be valid and should also be reusable on the server side.
Summing it up: On this side, in the stub definition, you can use patterns for request stubbing and you need exact values for responses.
Server Side
Being a service Y since you are developing your stub, you need to be sure that it’s actually resembling your concrete implementation. You can’t have a situation where your stub acts in one way and your application on production behaves in a different way.
That’s why from the provided stub acceptance tests will be generated that will ensure that your application behaves in the same way as you define in your stub.
Summing it up: On this side, in the stub definition, you need exact values as request and can use patterns/methods for response verification.
Dependencies
Accurest and Stub Runner are using the following libraries
Additional links
Below you can find some resources related to Accurest and Stub Runner. Note that some can be outdated since the Accurest project is under constant development.
Videos
Olga Maciaszek-Sharma talking about Accurest
Marcin Grzejszczak and Jakub Kubryński talking about Accurest
Samples
Here you can find some working samples. Check the readme of each project for more information.
Contract DSL
Contract DSL in Accurest is written in Groovy, but don’t be alarmed if you didn’t use Groovy before. Knowledge of the language is not really needed as our DSL uses only a tiny subset of it (namely literals, method calls and closures). What’s more, Accurest’s DSL is designed to be programmer-readable without any knowledge of the DSL itself - it’s statically typed.
Since 1.1.0 you can use the io.codearte.accurest.dsl.Accurest class in your DSL files.
|
Let’s look at full example of a contract definition.
io.codearte.accurest.dsl.GroovyDsl.make {
request {
method 'PUT'
url '/api/12'
headers {
header 'Content-Type': 'application/vnd.com.ofg.twitter-places-analyzer.v1+json'
}
body '''\
[{
"created_at": "Sat Jul 26 09:38:57 +0000 2014",
"id": 492967299297845248,
"id_str": "492967299297845248",
"text": "Gonna see you at Warsaw",
"place":
{
"attributes":{},
"bounding_box":
{
"coordinates":
[[
[-77.119759,38.791645],
[-76.909393,38.791645],
[-76.909393,38.995548],
[-77.119759,38.995548]
]],
"type":"Polygon"
},
"country":"United States",
"country_code":"US",
"full_name":"Washington, DC",
"id":"01fbe706f872cb32",
"name":"Washington",
"place_type":"city",
"url": "http://api.twitter.com/1/geo/id/01fbe706f872cb32.json"
}
}]
'''
}
response {
status 200
}
}
Not all features of the DSL are used in example above. If you didn’t find what you are looking for, please check next paragraphs on this page.
You can easily compile Accurest Contracts to WireMock stubs mapping using standalone maven command:
mvn io.codearte.accurest:accurest-maven-plugin:convert
.
Limitations
Accurest doesn’t support XML properly. Please use JSON or help us implement this feature. |
Accurest supports equality check on text response. Regular expressions are not yet available. |
HTTP Top-Level Elements
Following methods can be called in the top-level closure of a contract definition. Request and response are mandatory, priority is optional.
io.codearte.accurest.dsl.GroovyDsl.make {
// Definition of HTTP request part of the contract
// (this can be a valid request or invalid depending
// on type of contract being specified).
request {
//...
}
// Definition of HTTP response part of the contract
// (a service implementing this contract should respond
// with following response after receiving request
// specified in "request" part above).
response {
//...
}
// Contract priority, which can be used for overriding
// contracts (1 is highest). Priority is optional.
priority 1
}
Request
HTTP protocol requires only method and address to be specified in a request. The same information is mandatory in request definition of Accurest contract.
io.codearte.accurest.dsl.GroovyDsl.make {
request {
// HTTP request method (GET/POST/PUT/DELETE).
method 'GET'
// Path component of request URL is specified as follows.
urlPath('/users')
}
response {
//...
}
}
It is possible to specify whole url
instead of just path, but urlPath
is the recommended way as it makes the tests host-independent.
io.codearte.accurest.dsl.GroovyDsl.make {
request {
method 'GET'
// Specifying `url` and `urlPath` in one contract is illegal.
url('http://localhost:8888/users')
}
response {
//...
}
}
Request may contain query parameters, which are specified in a closure nested in a call to urlPath
or url
.
io.codearte.accurest.dsl.GroovyDsl.make {
request {
//...
urlPath('/users') {
// Each parameter is specified in form
// `'paramName' : paramValue` where parameter value
// may be a simple literal or one of matcher functions,
// all of which are used in this example.
queryParameters {
// If a simple literal is used as value
// default matcher function is used (equalTo)
parameter 'limit': 100
// `equalTo` function simply compares passed value
// using identity operator (==).
parameter 'filter': equalTo("email")
// `containing` function matches strings
// that contains passed substring.
parameter 'gender': value(stub(containing("[mf]")), server('mf'))
// `matching` function tests parameter
// against passed regular expression.
parameter 'offset': value(stub(matching("[0-9]+")), server(123))
// `notMatching` functions tests if parameter
// does not match passed regular expression.
parameter 'loginStartsWith': value(stub(notMatching(".{0,2}")), server(3))
}
}
//...
}
response {
//...
}
}
It may contain additional request headers…
io.codearte.accurest.dsl.GroovyDsl.make {
request {
//...
// Each header is added in form `'Header-Name' : 'Header-Value'`.
headers {
header 'Content-Type': 'application/json'
}
//...
}
response {
//...
}
}
…and a request body.
io.codearte.accurest.dsl.GroovyDsl.make {
request {
//...
// JSON and XML formats of request body are supported.
// Format will be determined from a header or body's content.
body '''{ "login" : "john", "name": "John The Contract" }'''
}
response {
//...
}
}
Body’s format can also be specified explicitly by invoking one of format functions.
io.codearte.accurest.dsl.GroovyDsl.make {
request {
//...
// In this case body will be formatted as XML.
body equalToXml(
'''<user><login>john</login><name>John The Contract</name></user>'''
)
}
response {
//...
}
}
Response
Minimal response must contain HTTP status code.
io.codearte.accurest.dsl.GroovyDsl.make {
request {
//...
}
response {
// Status code sent by the server
// in response to request specified above.
status 200
}
}
Besides status response may contain headers and body, which are specified the same way as in the request (see previous paragraph).
Regular expressions
You can use regular expressions to write your requests in Contract DSL. It is particularly useful when you want to indicate that a given response should be provided for requests that follow a given pattern. Also, you can use it when you need to use patterns and not exact values both for your test and your server side tests.
Please see the example below:
io.codearte.accurest.dsl.GroovyDsl.make {
request {
method('GET')
url $(client(~/\/[0-9]{2}/), server('/12'))
}
response {
status 200
body(
id: value(
client('123'),
server(regex('[0-9]+'))
),
surname: $(
client('Kowalsky'),
server('Lewandowski')
),
name: 'Jan',
created: $(client('2014-02-02 12:23:43'), server(execute('currentDate(it)'))),
correlationId: value(client('5d1f9fef-e0dc-4f3d-a7e4-72d2220dd827'),
server(regex('[a-fA-F0-9]{8}-[a-fA-F0-9]{4}-[a-fA-F0-9]{4}-[a-fA-F0-9]{4}-[a-fA-F0-9]{12}'))
)
)
headers {
header 'Content-Type': 'text/plain'
}
}
}
Passing optional parameters
It is possible to provide optional parameters in your contract. It’s only possible to have optional parameter for the:
-
STUB side of the Request
-
TEST side of the Response
Example:
io.codearte.accurest.dsl.GroovyDsl.make {
priority 1
request {
method 'POST'
url '/users/password'
headers {
header 'Content-Type': 'application/json'
}
body(
email: $(stub(optional(regex(email()))), test('abc@abc.com')),
callback_url: $(stub(regex(hostname())), test('http://partners.com'))
)
}
response {
status 404
headers {
header 'Content-Type': 'application/json'
}
body(
code: value(stub("123123"), test(optional("123123")))
)
}
}
By wrapping a part of the body with the optional()
method you are in fact creating a regular expression that should be present 0 or more times.
That way for the example above the following test would be generated if you pick Spock:
"""
given:
def request = given()
.header('Content-Type', 'application/json')
.body('''{"email":"abc@abc.com","callback_url":"http://partners.com"}''')
when:
def response = given().spec(request)
.post("/users/password")
then:
response.statusCode == 404
response.header('Content-Type') == 'application/json'
and:
DocumentContext parsedJson = JsonPath.parse(response.body.asString())
assertThatJson(parsedJson).field("code").matches("(123123)?")
"""
and the following stub:
'''
{
"request" : {
"url" : "/users/password",
"method" : "POST",
"bodyPatterns" : [ {
"matchesJsonPath" : "$[?(@.email =~ /([a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\\\.[a-zA-Z]{2,4})?/)]"
}, {
"matchesJsonPath" : "$[?(@.callback_url =~ /((http[s]?|ftp):\\\\/)\\\\/?([^:\\\\/\\\\s]+)(:[0-9]{1,5})?/)]"
} ],
"headers" : {
"Content-Type" : {
"equalTo" : "application/json"
}
}
},
"response" : {
"status" : 404,
"body" : "{\\"code\\":\\"123123\\",\\"message\\":\\"User not found by email == [not.existing@user.com]\\"}",
"headers" : {
"Content-Type" : "application/json"
}
},
"priority" : 1
}
'''
Executing custom methods on server side
It is also possible to define a method call to be executed on the server side during the test. Such a method can be added to the class defined as "baseClassForTests" in the configuration. Please see the examples below:
Groovy DSL
io.codearte.accurest.dsl.GroovyDsl.make {
request {
method 'PUT'
url $(client(regex('^/api/[0-9]{2}$')), server('/api/12'))
headers {
header 'Content-Type': 'application/json'
}
body '''\
[{
"text": "Gonna see you at Warsaw"
}]
'''
}
response {
body (
path: $(client('/api/12'), server(regex('^/api/[0-9]{2}$'))),
correlationId: $(client('1223456'), server(execute('isProperCorrelationId($it)')))
)
status 200
}
}
Base Mock Spec
abstract class BaseMockMvcSpec extends Specification {
def setup() {
RestAssuredMockMvc.standaloneSetup(new PairIdController())
}
void isProperCorrelationId(Integer correlationId) {
assert correlationId == 123456
}
void isEmpty(String value) {
assert value == null
}
}
JAX-RS support
Starting with release 0.8.0 we support JAX-RS 2 Client API. Base class needs to define protected WebTarget webTarget
and server initialization, right now the only option how to test JAX-RS API is to start a web server.
Request with a body needs to have a content type set otherwise application/octet-stream
is going to be used.
In order to use JAX-RS mode, use the following settings:
testMode == 'JAXRSCLIENT'
Example of a test API generated:
'''
// when:
Response response = webTarget
.path("/users")
.queryParam("limit", "10")
.queryParam("offset", "20")
.queryParam("filter", "email")
.queryParam("sort", "name")
.queryParam("search", "55")
.queryParam("age", "99")
.queryParam("name", "Denis.Stepanov")
.queryParam("email", "bob@email.com")
.request()
.method("GET");
String responseAsString = response.readEntity(String.class);
// then:
assertThat(response.getStatus()).isEqualTo(200);
// and:
DocumentContext parsedJson = JsonPath.parse(responseAsString);
assertThatJson(parsedJson).field("property1").isEqualTo("a");
'''
Messaging Top-Level Elements
Feature available since 1.1.0 |
The DSL for messaging looks a little bit different than the one that focuses on HTTP.
Output triggered by a method
The output message can be triggered by calling a method (e.g. a Scheduler was started and a message was sent)
def dsl = GroovyDsl.make {
// Human readable description
description 'Some description'
// Label by means of which the output message can be triggered
label 'some_label'
// input to the contract
input {
// the contract will be triggered by a method
triggeredBy('bookReturnedTriggered()')
}
// output message of the contract
outputMessage {
// destination to which the output message will be sent
sentTo('output')
// the body of the output message
body('''{ "bookName" : "foo" }''')
// the headers of the output message
headers {
header('BOOK-NAME', 'foo')
}
}
}
In this case the output message will be sent to output
if a method called bookReturnedTriggered
will be executed. In the message publisher’s side
we will generate a test that will call that method to trigger the message. On the consumer side you can use the some_label
to trigger the message.
Output triggered by a message
The output message can be triggered by receiving a message.
def dsl = GroovyDsl.make {
description 'Some Description'
label 'some_label'
// input is a message
input {
// the message was received from this destination
messageFrom('input')
// has the following body
messageBody([
bookName: 'foo'
])
// and the following headers
messageHeaders {
header('sample', 'header')
}
}
outputMessage {
sentTo('output')
body([
bookName: 'foo'
])
headers {
header('BOOK-NAME', 'foo')
}
}
}
In this case the output message will be sent to output
if a proper message will be received on the input
destination. In the message publisher’s side
we will generate a test that will send the input message to the defined destination. On the consumer side you can either send a message to the input
destination or use the some_label
to trigger the message.
Consumer / Producer
In HTTP you have a notion of client
/stub and `server
/test
notation. You can use them also in messaging but we’re providing also the consumer
and produer
methods
as presented below (note you can use either $
or value
methods to provide consumer
and producer
parts)
Accurest.make {
label 'some_label'
input {
messageFrom value(consumer('jms:output'), producer('jms:input'))
messageBody([
bookName: 'foo'
])
messageHeaders {
header('sample', 'header')
}
}
outputMessage {
sentTo $(consumer('jms:input'), producer('jms:output'))
body([
bookName: 'foo'
])
}
}
Accurest HTTP
Gradle Project
Prerequisites
In order to use Accurest with Wiremock you have to use gradle or maven plugin.
Add gradle plugin
buildscript {
repositories {
mavenCentral()
}
dependencies {
classpath 'io.codearte.accurest:accurest-gradle-plugin:${accurest_version}'
}
}
apply plugin: 'groovy'
apply plugin: 'accurest'
dependencies {
testCompile 'org.codehaus.groovy:groovy-all:2.4.6'
testCompile 'org.spockframework:spock-core:1.0-groovy-2.4'
testCompile 'com.jayway.restassured:spring-mock-mvc:2.9.0' // needed if you're going to use Spring MockMvc
}
Add maven plugin
<plugin>
<groupId>io.codearte.accurest</groupId>
<artifactId>accurest-maven-plugin</artifactId>
<executions>
<execution>
<goals>
<goal>convert</goal>
<goal>generateStubs</goal>
<goal>generateTests</goal>
</goals>
</execution>
</executions>
</plugin>
Read more: accurest-maven-plugin
Add stubs
By default Accurest is looking for stubs in src/test/resources/accurest
directory.
Directory containing stub definitions is treated as a class name, and each stub definition is treated as a single test. We assume that it contains at least one directory which will be used as test class name. If there is more than one level of nested directories all except the last one will be used as package name. So with following structure
src/test/resources/accurest/myservice/shouldCreateUser.groovy
src/test/resources/accurest/myservice/shouldReturnUser.groovy
Accurest will create test class defaultBasePackage.MyService
with two methods
-
shouldCreateUser()
-
shouldReturnUser()
Run plugin
Plugin registers itself to be invoked before check
task. You have nothing to do as long as you want it to be part of your build process. If you just want to generate tests please invoke generateAccurest
task.
Configure plugin
To change default configuration just add accurest
snippet to your Gradle config
accurest {
testMode = 'MockMvc'
baseClassForTests = 'org.mycompany.tests'
generatedTestSourcesDir = project.file('src/accurest')
}
Configuration options
-
testMode - defines mode for acceptance tests. By default MockMvc which is based on Spring’s MockMvc. It can also be changed to JaxRsClient or to Explicit for real HTTP calls.
-
imports - array with imports that should be included in generated tests (for example ['org.myorg.Matchers']). By default empty array []
-
staticImports - array with static imports that should be included in generated tests(for example ['org.myorg.Matchers.*']). By default empty array []
-
basePackageForTests - specifies base package for all generated tests. By default set to io.codearte.accurest.tests
-
baseClassForTests - base class for generated tests. By default
spock.lang.Specification
if using Spock tests. -
ruleClassForTests - specifies Rule which should be added to generated test classes.
-
ignoredFiles - Ant matcher allowing defining stub files for which processing should be skipped. By default empty array []
-
contractsDslDir - directory containing contracts written using the GroovyDSL. By default
$rootDir/src/test/resources/accurest
-
generatedTestSourcesDir - test source directory where tests generated from Groovy DSL should be placed. By default
$buildDir/generated-test-sources/accurest
-
stubsOutputDir - dir where the generated Wiremock stubs from Groovy DSL should be placed
-
targetFramework - the target test framework to be used; currently Spock and JUnit are supported with JUnit being the default framework
Base class for tests
When using Accurest in default MockMvc you need to create a base specification for all generated acceptance tests. In this class you need to point to endpoint which should be verified.
abstract class BaseMockMvcSpec extends Specification {
def setup() {
RestAssuredMockMvc.standaloneSetup(new PairIdController())
}
void isProperCorrelationId(Integer correlationId) {
assert correlationId == 123456
}
void isEmpty(String value) {
assert value == null
}
}
In case of using Explicit
mode, you can use base class to initialize the whole tested app similarly as in regular integration tests. In case of JAXRSCLIENT
mode this base class
should also contain protected WebTarget webTarget
field, right now the only option to test JAX-RS API is to start a web server.
Invoking generated tests
To ensure that provider side is complaint with defined contracts, you need to invoke:
./gradlew generateAccurest test
Accurest on consumer side
In consumer service you need to configure Accurest plugin in exactly the same way as in case of provider. If you don’t want to use Stub Runner then you need to copy contracts stored in
src/test/resources/accurest
and generate WireMock json stubs using:
./gradlew generateWireMockClientStubs
Note that stubsOutputDir
option has to be set for stub generation to work.
When present, json stubs can be used in consumer automated tests.
@ContextConfiguration(loader == SpringApplicationContextLoader, classes == Application)
class LoanApplicationServiceSpec extends Specification {
@ClassRule
@Shared
WireMockClassRule wireMockRule == new WireMockClassRule()
@Autowired
LoanApplicationService sut
def 'should successfully apply for loan'() {
given:
LoanApplication application =
new LoanApplication(client: new Client(pesel: '12345678901'), amount: 123.123)
when:
LoanApplicationResult loanApplication == sut.loanApplication(application)
then:
loanApplication.loanApplicationStatus === LoanApplicationStatus.LOAN_APPLIED
loanApplication.rejectionReason === null
}
}
Underneath LoanApplication makes a call to FraudDetection service. This request is handled by Wiremock server configured using stubs generated by Accurest.
Using in your Maven project
Add maven plugin
<plugin>
<groupId>io.codearte.accurest</groupId>
<artifactId>accurest-maven-plugin</artifactId>
<executions>
<execution>
<goals>
<goal>convert</goal>
<goal>generateStubs</goal>
<goal>generateTests</goal>
</goals>
</execution>
</executions>
</plugin>
Read more: accurest-maven-plugin
Add stubs
By default Accurest is looking for stubs in src/test/resources/accurest
directory.
Directory containing stub definitions is treated as a class name, and each stub definition is treated as a single test.
We assume that it contains at least one directory which will be used as test class name. If there is more than one level of nested directories all except the last one will be used as package name.
So with following structure
src/test/resources/accurest/myservice/shouldCreateUser.groovy
src/test/resources/accurest/myservice/shouldReturnUser.groovy
Accurest will create test class defaultBasePackage.MyService
with two methods
- shouldCreateUser()
- shouldReturnUser()
Run plugin
Plugin goal generateTests
is assigned to be invoked in phase generate-test-sources
. You have nothing to do as long as you want it to be part of your build process. If you just want to generate tests please invoke generateTests
goal.
Configure plugin
To change default configuration just add configuration
section to plugin definition or execution
definition.
<plugin>
<groupId>io.codearte.accurest</groupId>
<artifactId>accurest-maven-plugin</artifactId>
<executions>
<execution>
<goals>
<goal>convert</goal>
<goal>generateStubs</goal>
<goal>generateTests</goal>
</goals>
</execution>
</executions>
<configuration>
<basePackageForTests>com.ofg.twitter.place</basePackageForTests>
<baseClassForTests>com.ofg.twitter.place.BaseMockMvcSpec</baseClassForTests>
</configuration>
</plugin>
Important configuration options
-
testMode - defines mode for acceptance tests. By default
MockMvc
which is based on Spring’s MockMvc. It can also be changed toJaxRsClient
or toExplicit
for real HTTP calls. -
basePackageForTests - specifies base package for all generated tests. By default set to
io.codearte.accurest.tests
. -
ruleClassForTests - specifies Rule which should be added to generated test classes.
-
baseClassForTests - base class for generated tests. By default
spock.lang.Specification
if using Spock tests. -
contractsDir - directory containing contracts written using the GroovyDSL. By default
/src/test/resources/accurest
. -
testFramework - the target test framework to be used; currently Spock and JUnit are supported with Spock being the default framework
For complete information take a look at Plugin Documentation
Base class for tests
When using Accurest in default MockMvc you need to create a base specification for all generated acceptance tests. In this class you need to point to endpoint which should be verified.
package org.mycompany.tests
import org.mycompany.ExampleSpringController
import com.jayway.restassured.module.mockmvc.RestAssuredMockMvc
import spock.lang.Specification
class MvcSpec extends Specification {
def setup() {
RestAssuredMockMvc.standaloneSetup(new ExampleSpringController())
}
}
In case of using Explicit
mode, you can use base class to initialize the whole tested app similarly as in regular integration tests. In case of JAXRSCLIENT
mode this base class should also contain protected WebTarget webTarget
field, right now the only option to test JAX-RS API is to start a web server.
Invoking generated tests
Accurest Maven Plugins generates verification code into directory /generated-test-sources/accurest
and attach this directory to testCompile
goal.
For Groovy Spock code use:
<plugin>
<groupId>org.codehaus.gmavenplus</groupId>
<artifactId>gmavenplus-plugin</artifactId>
<version>1.5</version>
<executions>
<execution>
<goals>
<goal>testCompile</goal>
</goals>
</execution>
</executions>
<configuration>
<testSources>
<testSource>
<directory>${project.basedir}/src/test/groovy</directory>
<includes>
<include>**/*.groovy</include>
</includes>
</testSource>
<testSource>
<directory>${project.build.directory}/generated-test-sources/accurest</directory>
<includes>
<include>**/*.groovy</include>
</includes>
</testSource>
</testSources>
</configuration>
</plugin>
To ensure that provider side is complaint with defined contracts, you need to invoke mvn generateTest test
Accurest on consumer side
In consumer service you need to configure Accurest plugin in exactly the same way as in case of provider. You need to copy contracts stored in src/test/resources/accurest
and generate Wiremock json stubs using: mvn generateStubs
command. By default generated WireMock mapping is stored in directory target/mappings
. Your project should create from this generated mappings additional artifact with classifier stubs
for easy deploy to maven repository.
Sample configuration:
<plugin>
<groupId>io.codearte.accurest</groupId>
<artifactId>accurest-maven-plugin</artifactId>
<version>${accurest-plugin.version}</version>
<executions>
<execution>
<goals>
<goal>convert</goal>
<goal>generateStubs</goal>
</goals>
</execution>
</executions>
</plugin>
When present, json stubs can be used in consumer automated tests.
@ContextConfiguration(loader == SpringApplicationContextLoader, classes == Application)
class LoanApplicationServiceSpec extends Specification {
@ClassRule
@Shared
WireMockClassRule wireMockRule == new WireMockClassRule()
@Autowired
LoanApplicationService sut
def 'should successfully apply for loan'() {
given:
LoanApplication application =
new LoanApplication(client: new Client(pesel: '12345678901'), amount: 123.123)
when:
LoanApplicationResult loanApplication == sut.loanApplication(application)
then:
loanApplication.loanApplicationStatus === LoanApplicationStatus.LOAN_APPLIED
loanApplication.rejectionReason === null
}
}
Underneath LoanApplication makes a call to FraudDetection service. This request is handled by Wiremock server configured using stubs generated by Accurest.
Scenarios
It’s possible to handle scenarios with Accurest. All you need to do is to stick to proper naming convention while creating your contracts. The convention requires to include order number followed by the underscore.
my_contracts_dir\
scenario1\
1_login.groovy
2_showCart.groovy
3_logout.groovy
Such tree will cause Accurest generating Wiremock’s scenario with name scenario1
and three steps:
- login marked as Started
pointing to:
- showCart marked as Step1
pointing to:
- logout marked as Step2
which will close the scenario.
More details about Wiremock scenarios can be found under [http://wiremock.org/stateful-behaviour.html](http://wiremock.org/stateful-behaviour.html)
Accurest will also generate tests with guaranteed order of execution.
Accurest Messaging
Feature available since 1.1.0 |
Accurest allows you to verify your application that uses messaging as means of communication. All of our integrations are working with Spring but you can also set one yourself.
Integrations
You can use one of the three integration configurations:
-
Apache Camel
-
Spring Integration
-
Spring Cloud Stream
If you’re using Spring Boot, the aforementioned test configurations will be appended automatically.
You have to provide as a dependency one of the Accurest Messaging modules. Example for Gradle:
// for Apache Camel
testCompile "io.codearte.accurest:accurest-messaging-camel:${accurestVersion}"
// for Spring Integration
testCompile "io.codearte.accurest:accurest-messaging-integration:${accurestVersion}"
// for Spring Cloud Stream
testCompile "io.codearte.accurest:accurest-messaging-stream:${accurestVersion}"
Manual Integration
The accurest-messaging-core
module contains 3 main interfaces:
-
AccurestMessage
- describes a message received / sent to a channel / queue / topic etc. -
AccurestMessageBuilder
- describes how to build a message -
AccurestMessaging
- class that allows you to build, send and receive messages -
AccurestFilter
- interface to filter out the messages that do not follow the pattern from the DSL
In the generated test the AccurestMessaging
is injected via @Inject
annotation thus you can use other injection
frameworks than Spring.
You have to provide as a dependency the accurest-messaging-core
module. Example for Gradle:
testCompile "io.codearte.accurest:accurest-messaging-core:${accurestVersion}"
Publisher side test generation
Having the input
or outputMessage
sections in your DSL will result in creation of tests on the publisher’s side. By default
JUnit tests will be created, however there is also a possibility to create Spock tests.
There are 3 main scenarios that we should take into consideration:
-
Scenario 1: there is no input message that produces an output one. The output message is triggered by a component inside the application (e.g. scheduler)
-
Scenario 2: the input message triggers an output message
-
Scenario 3: the input message is consumed and there is no output message
Scenario 1 (no input message)
For the given contract:
def contractDsl = GroovyDsl.make {
label 'some_label'
input {
triggeredBy('bookReturnedTriggered()')
}
outputMessage {
sentTo('activemq:output')
body('''{ "bookName" : "foo" }''')
headers {
header('BOOK-NAME', 'foo')
}
}
}
The following JUnit test will be created:
'''
// when:
bookReturnedTriggered();
// then:
AccurestMessage response = accurestMessaging.receiveMessage("activemq:output");
assertThat(response).isNotNull();
assertThat(response.getHeader("BOOK-NAME")).isEqualTo("foo");
// and:
DocumentContext parsedJson = JsonPath.parse(accurestObjectMapper.writeValueAsString(response.getPayload()));
assertThatJson(parsedJson).field("bookName").isEqualTo("foo");
'''
And the following Spock test would be created:
'''
when:
bookReturnedTriggered()
then:
def response = accurestMessaging.receiveMessage('activemq:output')
assert response != null
response.getHeader('BOOK-NAME') == 'foo'
and:
DocumentContext parsedJson = JsonPath.parse(accurestObjectMapper.writeValueAsString(response.payload))
assertThatJson(parsedJson).field("bookName").isEqualTo("foo")
'''
Scenario 2 (output triggered by input)
For the given contract:
def contractDsl = GroovyDsl.make {
label 'some_label'
input {
messageFrom('jms:input')
messageBody([
bookName: 'foo'
])
messageHeaders {
header('sample', 'header')
}
}
outputMessage {
sentTo('jms:output')
body([
bookName: 'foo'
])
headers {
header('BOOK-NAME', 'foo')
}
}
}
The following JUnit test will be created:
'''
// given:
AccurestMessage inputMessage = accurestMessaging.create(
"{\\"bookName\\":\\"foo\\"}"
, headers()
.header("sample", "header"));
// when:
accurestMessaging.send(inputMessage, "jms:input");
// then:
AccurestMessage response = accurestMessaging.receiveMessage("jms:output");
assertThat(response).isNotNull();
assertThat(response.getHeader("BOOK-NAME")).isEqualTo("foo");
// and:
DocumentContext parsedJson = JsonPath.parse(accurestObjectMapper.writeValueAsString(response.getPayload()));
assertThatJson(parsedJson).field("bookName").isEqualTo("foo");
'''
And the following Spock test would be created:
"""\
given:
def inputMessage = accurestMessaging.create(
'''{"bookName":"foo"}''',
['sample': 'header']
)
when:
accurestMessaging.send(inputMessage, 'jms:input')
then:
def response = accurestMessaging.receiveMessage('jms:output')
assert response !- null
response.getHeader('BOOK-NAME') == 'foo'
and:
DocumentContext parsedJson = JsonPath.parse(accurestObjectMapper.writeValueAsString(response.payload))
assertThatJson(parsedJson).field("bookName").isEqualTo("foo")
"""
Scenario 3 (no output message)
For the given contract:
def contractDsl = GroovyDsl.make {
label 'some_label'
input {
messageFrom('jms:delete')
messageBody([
bookName: 'foo'
])
messageHeaders {
header('sample', 'header')
}
assertThat('bookWasDeleted()')
}
}
The following JUnit test will be created:
'''
// given:
AccurestMessage inputMessage = accurestMessaging.create(
"{\\"bookName\\":\\"foo\\"}"
, headers()
.header("sample", "header"));
// when:
accurestMessaging.send(inputMessage, "jms:delete");
// then:
bookWasDeleted();
'''
And the following Spock test would be created:
'''
given:
def inputMessage = accurestMessaging.create(
\'\'\'{"bookName":"foo"}\'\'\',
['sample': 'header']
)
when:
accurestMessaging.send(inputMessage, 'jms:delete')
then:
noExceptionThrown()
bookWasDeleted()
'''
Consumer Stub Side generation
Unlike the HTTP part - in Messaging we need to publish the Groovy DSL inside the JAR with a stub. Then it’s parsed on the consumer side and proper stubbed routes are created.
For more infromation please consult the Stub Runner Messaging sections.
Gradle Setup
Example of Accurest Gradle setup:
ext {
contractsDir = file("mappings")
stubsOutputDirRoot = file("${project.buildDir}/production/${project.name}-stubs/")
wireMockStubsOutputDir = file(new File(stubsOutputDirRoot, 'repository/mappings/'))
contractsOutputDir = file(new File(stubsOutputDirRoot, 'repository/accurest/'))
}
task copyContracts(type: Copy) {
from contractsDir
include '**/*.groovy'
into contractsOutputDir
}
task stubsJar(type: Jar, dependsOn: ["generateWireMockClientStubs", copyContracts]) {
baseName = "${project.name}"
classifier = "stubs"
from stubsOutputDirRoot
}
artifacts {
archives stubsJar
}
publishing {
publications {
stubs(MavenPublication) {
artifactId "${project.name}-stubs"
artifact stubsJar
}
}
}
Maven Setup
Example of Maven can be found in the Accurest Maven Plugin README
Stub Runner
One of the issues that you could have encountered while using Accurest was to pass the generated WireMock JSON stubs from the server side to the client side (or various clients). The same takes place in terms of client side generation for messaging.
Copying the JSON files / setting the client side for messaging manually is out of the question.
Publishing stubs as JARs
The easiest approach would be to centralize the way stubs are kept. For example you can keep them as JARs in a Maven repository.
Gradle
Example of Accurest Gradle setup:
ext {
contractsDir = file("mappings")
stubsOutputDirRoot = file("${project.buildDir}/production/${project.name}-stubs/")
wireMockStubsOutputDir = file(new File(stubsOutputDirRoot, 'repository/mappings/'))
contractsOutputDir = file(new File(stubsOutputDirRoot, 'repository/accurest/'))
}
task copyContracts(type: Copy) {
from contractsDir
include '**/*.groovy'
into contractsOutputDir
}
task stubsJar(type: Jar, dependsOn: ["generateWireMockClientStubs", copyContracts]) {
baseName = "${project.name}"
classifier = "stubs"
from stubsOutputDirRoot
}
artifacts {
archives stubsJar
}
publishing {
publications {
stubs(MavenPublication) {
artifactId "${project.name}-stubs"
artifact stubsJar
}
}
}
Maven
Example of Maven can be found in the Accurest Maven Plugin README
Modules
Accurest comes with a new structure of modules
└── stub-runner
├── stub-runner
├── stub-runner-boot
├── stub-runner-junit
├── stub-runner-spring
└── stub-runner-spring-cloud
Stub Runner Core
Runs stubs for service collaborators. Treating stubs as contracts of services allows to use stub-runner as an implementation of Consumer Driven Contracts.
Stub Runner allows you to automatically download the stubs of the provided dependencies, start WireMock servers for them and feed them with proper stub definitions. For messaging, special stub routes are defined.
Running stubs
Running using main app
You can set the following options to the main class:
-maxp (--maxPort) N : Maximum port value to be assigned to the
Wiremock instance. Defaults to 15000
(default: 15000)
-minp (--minPort) N : Minimal port value to be assigned to the
Wiremock instance. Defaults to 10000
(default: 10000)
-s (--stubs) VAL : Comma separated list of Ivy representation of
jars with stubs. Eg. groupid:artifactid1,group
id2:artifactid2:version:classifier
-sr (--stubRepositoryRoot) VAL : Location of a Jar containing server where you
keep your stubs (e.g. http://nexus.net/content
/repositories/repository)
-ss (--stubsSuffix) VAL : Suffix for the jar containing stubs (e.g.
'stubs' if the stub jar would have a 'stubs'
classifier for stubs: foobar-stubs ).
Defaults to 'stubs' (default: stubs)
-wo (--workOffline) : Switch to work offline. Defaults to 'false'
(default: false)
Building a Fat Jar
Just call the following command:
./gradlew stub-runner-root:stub-runner:shadowJar -PfatJar
and inside the build/lib
there will be a Fat Jar with classifier fatJar
waiting for you to execute. E.g.
java -jar stub-runner/stub-runner/build/libs/stub-runner-1.0.1-SNAPSHOT-fatJar.jar -sr http://a.b.com -s a:b:c,d:e,f:g:h:i
Stub runner configuration
You can configure the stub runner by either passing the full arguments list with the -Pargs
like this:
./gradlew stub-runner-root:stub-runner:run -Pargs="-c pl -minp 10000 -maxp 10005 -s a:b:c,d:e,f:g:h"
or each parameter separately with a -P
prefix and without the hyphen -
in the name of the param
./gradlew stub-runner-root:stub-runner:run -Pc=pl -Pminp=10000 -Pmaxp=10005 -Ps=a:b:c,d:e,f:g:h
HTTP Stubs
Stubs are defined in JSON documents, whose syntax is defined in WireMock documentation
Example:
{
"request": {
"method": "GET",
"url": "/ping"
},
"response": {
"status": 200,
"body": "pong",
"headers": {
"Content-Type": "text/plain"
}
}
}
Viewing registered mappings
Every stubbed collaborator exposes list of defined mappings under __/admin/
endpoint.
Messaging Stubs
Depending on the provided Stub Runner dependency and the DSL the messaging routes are automatically set up.
Stub Runner Boot
Feature available since 1.1.0 |
Accurest Stub Runner Boot is a Spring Boot application that exposes REST endpoints to trigger the messaging labels and to access started WireMock servers.
One of the usecases is to run some smoke (end to end) tests on a deployed application. You can read more about this in the "Microservice Deployment" article at Too Much Coding blog.
How to use it?
Just add the
compile "io.codearte.accurest:stub-runner-boot:${accurestVersion}"
and a messaging implementation:
// for Apache Camel
compile "io.codearte.accurest:stub-runner-messaging-camel:${accurestVersion}"
// for Spring Integration
compile "io.codearte.accurest:stub-runner-messaging-integration:${accurestVersion}"
// for Spring Cloud Stream
compile "io.codearte.accurest:stub-runner-messaging-stream:${accurestVersion}"
Build a fat-jar and you’re ready to go!
For the properties check the Stub Runner Spring section.
Endpoints
HTTP
-
GET
/stubs
- returns a list of all running stubs inivy:integer
notation -
GET
/stubs/{ivy}
- returns a port for the givenivy
notation (when calling the endpointivy
can also beartifactId
only)
Messaging
For Messaging
-
GET
/triggers
- returns a list of all running labels inivy : [ label1, label2 …]
notation -
POST
/triggers/{label}
- executes a trigger withlabel
-
POST
/triggers/{ivy}/{label}
- executes a trigger withlabel
for the givenivy
notation (when calling the endpointivy
can also beartifactId
only)
Example
@ContextConfiguration(classes = [StubRunnerBootSpec, StubRunnerBoot], loader = SpringApplicationContextLoader)
@EnableBinding
@Configuration
class StubRunnerBootSpec extends Specification {
@Autowired StubRunning stubRunning
def setup() {
RestAssuredMockMvc.standaloneSetup(new HttpStubsController(stubRunning),
new TriggerController(stubRunning))
}
def 'should return a list of running stub servers in "full ivy:port" notation'() {
when:
String response = RestAssuredMockMvc.get('/stubs').body.asString()
then:
def root = new JsonSlurper().parseText(response)
root.'io.codearte.accurest.stubs:streamService:0.0.1-SNAPSHOT:stubs' instanceof Integer
}
def 'should return a port on which a [#stubId] stub is running'() {
when:
def response = RestAssuredMockMvc.get("/stubs/${stubId}")
then:
response.statusCode == 200
response.body.as(Integer) > 0
where:
stubId << ['io.codearte.accurest.stubs:streamService:+:stubs',
'io.codearte.accurest.stubs:streamService:0.0.1-SNAPSHOT:stubs',
'io.codearte.accurest.stubs:streamService:+',
'io.codearte.accurest.stubs:streamService',
'streamService']
}
def 'should return 404 when missing stub was called'() {
when:
def response = RestAssuredMockMvc.get("/stubs/a:b:c:d")
then:
response.statusCode == 404
}
def 'should return a list of messaging labels that can be triggered when version and classifier are passed'() {
when:
String response = RestAssuredMockMvc.get('/triggers').body.asString()
then:
def root = new JsonSlurper().parseText(response)
root.'io.codearte.accurest.stubs:streamService:0.0.1-SNAPSHOT:stubs'?.containsAll(["delete_book","return_book_1","return_book_2"])
}
def 'should trigger a messaging label'() {
given:
StubRunning stubRunning = Mock()
RestAssuredMockMvc.standaloneSetup(new HttpStubsController(stubRunning), new TriggerController(stubRunning))
when:
def response = RestAssuredMockMvc.post("/triggers/delete_book")
then:
response.statusCode == 200
and:
1 * stubRunning.trigger('delete_book')
}
def 'should trigger a messaging label for a stub with [#stubId] ivy notation'() {
given:
StubRunning stubRunning = Mock()
RestAssuredMockMvc.standaloneSetup(new HttpStubsController(stubRunning), new TriggerController(stubRunning))
when:
def response = RestAssuredMockMvc.post("/triggers/$stubId/delete_book")
then:
response.statusCode == 200
and:
1 * stubRunning.trigger(stubId, 'delete_book')
where:
stubId << ['io.codearte.accurest.stubs:streamService:stubs', 'io.codearte.accurest.stubs:streamService', 'streamService']
}
def 'should return when trigger is missing'() {
when:
def response = RestAssuredMockMvc.post("/triggers/missing_label")
then:
response.statusCode == 404
def root = new JsonSlurper().parseText(response.body.asString())
root.'io.codearte.accurest.stubs:streamService:0.0.1-SNAPSHOT:stubs'?.containsAll(["delete_book","return_book_1","return_book_2"])
}
}
Stub Runner JUnit Rule
Stub Runner comes with a JUnit rule thanks to which you can very easily download and run stubs for given group and artifact id:
@ClassRule public static AccurestRule rule = new AccurestRule()
.repoRoot(repoRoot())
.downloadStub("io.codearte.accurest.stubs", "loanIssuance")
.downloadStub("io.codearte.accurest.stubs:fraudDetectionServer");
After that rule gets executed Stub Runner connects to your Maven repository and for the given list of dependencies tries to:
-
download them
-
cache them locally
-
unzip them to a temporary folder
-
start a WireMock server for each Maven dependency on a random port from the provided range of ports / provided port
-
feed the WireMock server with all JSON files that are valid WireMock definitions
Stub Runner uses Eclipse Aether mechanism to download the Maven dependencies. Check their docs for more information.
Since the AccurestRule
implements the StubFinder
it allows you to find the started stubs:
package io.codearte.accurest.stubrunner
import io.codearte.accurest.dsl.GroovyDsl
interface StubFinder extends StubTrigger {
/**
* For the given groupId and artifactId tries to find the matching
* URL of the running stub.
*
* @param groupId - might be null. In that case a search only via artifactId takes place
* @return URL of a running stub or null if not found
*/
URL findStubUrl(String groupId, String artifactId)
/**
* For the given Ivy notation {@code groupId:artifactId} tries to find the matching
* URL of the running stub. You can also pass only {@code artifactId}.
*
* @param ivyNotation - Ivy representation of the Maven artifact
* @return URL of a running stub or null if not found
*/
URL findStubUrl(String ivyNotation)
/**
* Returns all running stubs
*/
RunningStubs findAllRunningStubs()
/**
* Returns the list of Accurest contracts
*/
Map<StubConfiguration, Collection<GroovyDsl>> getAccurestContracts()
}
Example of usage in Spock tests:
@ClassRule @Shared AccurestRule rule = new AccurestRule()
.repoRoot(AccurestRuleSpec.getResource("/m2repo").toURI().toString())
.downloadStub("io.codearte.accurest.stubs", "loanIssuance")
.downloadStub("io.codearte.accurest.stubs:fraudDetectionServer")
def 'should start WireMock servers'() {
expect: 'WireMocks are running'
rule.findStubUrl('io.codearte.accurest.stubs', 'loanIssuance') != null
rule.findStubUrl('loanIssuance') != null
rule.findStubUrl('loanIssuance') == rule.findStubUrl('io.codearte.accurest.stubs', 'loanIssuance')
rule.findStubUrl('io.codearte.accurest.stubs:fraudDetectionServer') != null
and:
rule.findAllRunningStubs().isPresent('loanIssuance')
rule.findAllRunningStubs().isPresent('io.codearte.accurest.stubs', 'fraudDetectionServer')
rule.findAllRunningStubs().isPresent('io.codearte.accurest.stubs:fraudDetectionServer')
and: 'Stubs were registered'
"${rule.findStubUrl('loanIssuance').toString()}/name".toURL().text == 'loanIssuance'
"${rule.findStubUrl('fraudDetectionServer').toString()}/name".toURL().text == 'fraudDetectionServer'
}
Example of usage in JUnit tests:
@Test
public void should_start_wiremock_servers() throws Exception {
// expect: 'WireMocks are running'
then(rule.findStubUrl("io.codearte.accurest.stubs", "loanIssuance")).isNotNull();
then(rule.findStubUrl("loanIssuance")).isNotNull();
then(rule.findStubUrl("loanIssuance")).isEqualTo(rule.findStubUrl("io.codearte.accurest.stubs", "loanIssuance"));
then(rule.findStubUrl("io.codearte.accurest.stubs:fraudDetectionServer")).isNotNull();
// and:
then(rule.findAllRunningStubs().isPresent("loanIssuance")).isTrue();
then(rule.findAllRunningStubs().isPresent("io.codearte.accurest.stubs", "fraudDetectionServer")).isTrue();
then(rule.findAllRunningStubs().isPresent("io.codearte.accurest.stubs:fraudDetectionServer")).isTrue();
// and: 'Stubs were registered'
then(httpGet(rule.findStubUrl("loanIssuance").toString() + "/name")).isEqualTo("loanIssuance");
then(httpGet(rule.findStubUrl("fraudDetectionServer").toString() + "/name")).isEqualTo("fraudDetectionServer");
}
Check the Common properties for JUnit and Spring for more information on how to apply global configuration of Stub Runner.
Providing fixed ports
You can also run your stubs on fixed ports. You can do it in two different ways. One is to pass it in the properties, and the other via fluent API of JUnit rule.
Fluent API
When using the AccurestRule
you can add a stub to download and then pass the port for the last downloaded stub.
@ClassRule public static AccurestRule rule = new AccurestRule()
.repoRoot(repoRoot())
.downloadStub("io.codearte.accurest.stubs", "loanIssuance")
.withPort(12345)
.downloadStub("io.codearte.accurest.stubs:fraudDetectionServer:12346");
You can see that for this example the following test is valid:
then(rule.findStubUrl("loanIssuance")).isEqualTo(URI.create("http://localhost:12345").toURL());
then(rule.findStubUrl("fraudDetectionServer")).isEqualTo(URI.create("http://localhost:12346").toURL());
Stub Runner Spring
Sets up Spring configuration of the Stub Runner project.
By providing a list of stubs inside your configuration file the Stub Runner automatically downloads and registers in WireMock the selected stubs.
If you want to find the URL of your stubbed dependency you can autowire the StubFinder
interface and use
its methods as presented below:
@ContextConfiguration(classes = Config, loader = SpringApplicationContextLoader)
class StubRunnerConfigurationSpec extends Specification {
@Autowired StubFinder stubFinder
def 'should start WireMock servers'() {
expect: 'WireMocks are running'
stubFinder.findStubUrl('io.codearte.accurest.stubs', 'loanIssuance') != null
stubFinder.findStubUrl('loanIssuance') != null
stubFinder.findStubUrl('loanIssuance') == stubFinder.findStubUrl('io.codearte.accurest.stubs', 'loanIssuance')
stubFinder.findStubUrl('io.codearte.accurest.stubs:fraudDetectionServer') != null
and:
stubFinder.findAllRunningStubs().isPresent('loanIssuance')
stubFinder.findAllRunningStubs().isPresent('io.codearte.accurest.stubs', 'fraudDetectionServer')
stubFinder.findAllRunningStubs().isPresent('io.codearte.accurest.stubs:fraudDetectionServer')
and: 'Stubs were registered'
"${stubFinder.findStubUrl('loanIssuance').toString()}/name".toURL().text == 'loanIssuance'
"${stubFinder.findStubUrl('fraudDetectionServer').toString()}/name".toURL().text == 'fraudDetectionServer'
}
@Configuration
@Import(StubRunnerConfiguration)
@EnableAutoConfiguration
static class Config {}
}
for the following configuration file:
stubrunner.stubs.repository.root: classpath:m2repo/repository/
stubrunner.stubs.ids: io.codearte.accurest.stubs:loanIssuance,io.codearte.accurest.stubs:fraudDetectionServer
Stub Runner Spring Cloud
Registers the stubs in the provided Service Discovery. It’s enough to add the jar
io.codearte.accurest:stub-runner-spring-cloud
and the Stub Runner autoconfiguration should be picked up.
Stubbing Service Discovery
The most important feature of Stub Runner Spring Cloud
is the fact that it’s stubbing
-
DiscoveryClient
-
Ribbon
ServerList
that means that regardles of the fact whether you’re using Zookeeper, Consul, Eureka or anything else, you don’t need that in your tests.
We’re starting WireMock instances of your dependencies and we’re telling your application whenever you’re using Feign
, load balanced RestTemplate
or DiscoveryClient
directly, to call those stubbed servers instead of calling the real Service Discovery tool.
Additional Configuration
You can match the artifactId of the stub with the name of your app by using the stubrunner.stubs.idsToServiceIds:
map.
You can disable Stub Runner Ribbon support by providing: stubrunner.cloud.ribbon.enabled
equal to false
You can disable Stub Runner support by providing: stubrunner.cloud.enabled
equal to false
Common properties for JUnit and Spring
Some of the properties that are repetitive can be set using system properties or property sources (for Spring). Here are their names with their default values:
Property name | Default value | Description |
---|---|---|
stubrunner.port.range.min |
10000 |
Minimal value of a port for a started WireMock with stubs |
stubrunner.port.range.max |
15000 |
Minimal value of a port for a started WireMock with stubs |
stubrunner.stubs.repository.root |
Comma separated list of Maven repo urls. If blank then will call the local maven repo |
|
stubrunner.stubs.classifier |
stubs |
Default classifier for the stub artifacts |
stubrunner.work-offline |
false |
If true then will not contact any remote repositories to download stubs |
stubrunner.stubs.ids |
Comma separated list of Ivy notation of stubs to download |
Stub runner stubs ids
You can provide the stubs to download via the stubrunner.stubs.ids
system property. They follow the following pattern:
groupId:artifactId:version:classifier:port
version
, classifier
and port
are optional.
-
If you don’t provide the
port
then a random one will be picked -
If you don’t provide the
classifier
then the default one will be taken. -
If you don’t provide the
version
then the+
will be passed and the latest one will be downloaded
Where port
means the port of the WireMock server.
Stub Runner for Messaging
Feature available since 1.1.0 |
Stub Runner has the functionality to run the published stubs in memory. It can integrate with the following frameworks out of the box
-
Spring Integration
-
Spring Cloud Stream
-
Apache Camel
It also provides points of entry to integrate with any other solution on the market.
Stub triggering
To trigger a message it’s enough to use the StubTigger
interface:
package io.codearte.accurest.stubrunner
interface StubTrigger {
/**
* Triggers an event by a given label for a given {@code groupid:artifactid} notation. You can use only {@code artifactId} too.
*
* Feature related to messaging.
*
* @return true - if managed to run a trigger
*/
boolean trigger(String ivyNotation, String labelName)
/**
* Triggers an event by a given label.
*
* Feature related to messaging.
*
* @return true - if managed to run a trigger
*/
boolean trigger(String labelName)
/**
* Triggers all possible events.
*
* Feature related to messaging.
*
* @return true - if managed to run a trigger
*/
boolean trigger()
/**
* Returns a mapping of ivy notation of a dependency to all the labels it has.
*
* Feature related to messaging.
*/
Map<String, Collection<String>> labels()
}
For convenience the StubFinder
interface extends StubTrigger
so it’s enough to use only one in your tests.
StubTrigger
gives you the following options to trigger a message:
Trigger by label
stubFinder.trigger('return_book_1')
Trigger by group and artifact ids
stubFinder.trigger('io.codearte.accurest.stubs:camelService', 'return_book_1')
Trigger by artifact ids
stubFinder.trigger('camelService', 'return_book_1')
Trigger all messages
stubFinder.trigger()
Stub Runner Messaging Camel
Accurest Stub Runner’s messaging module gives you an easy way to integrate with Apache Camel. For the provided artifacts it will automatically download the stubs and register the required routes.
Adding it to the project
To use it you have to add the following dependency to your project (example for Gradle):
testCompile "io.codearte.accurest:stub-runner-messaging-camel:${accurestVersion}"
Examples
Stubs structure
Let us assume that we have the following Maven repository with a deployed stubs for the
camelService
application.
└── .m2
└── repository
└── io
└── codearte
└── accurest
└── stubs
└── camelService
├── 0.0.1-SNAPSHOT
│ ├── camelService-0.0.1-SNAPSHOT.pom
│ ├── camelService-0.0.1-SNAPSHOT-stubs.jar
│ └── maven-metadata-local.xml
└── maven-metadata-local.xml
And the stubs contain the following structure:
├── META-INF
│ └── MANIFEST.MF
└── repository
├── accurest
│ ├── bookDeleted.groovy
│ ├── bookReturned1.groovy
│ └── bookReturned2.groovy
└── mappings
Let’s consider the following contracts (let' number it with 1):
io.codearte.accurest.dsl.GroovyDsl.make {
label 'return_book_1'
input {
triggeredBy('bookReturnedTriggered()')
}
outputMessage {
sentTo('jms:output')
body('''{ "bookName" : "foo" }''')
headers {
header('BOOK-NAME', 'foo')
}
}
}
and number 2
io.codearte.accurest.dsl.GroovyDsl.make {
label 'return_book_2'
input {
messageFrom('jms:input')
messageBody([
bookName: 'foo'
])
messageHeaders {
header('sample', 'header')
}
}
outputMessage {
sentTo('jms:output')
body([
bookName: 'foo'
])
headers {
header('BOOK-NAME', 'foo')
}
}
}
Scenario 1 (no input message)
So as to trigger a message via the return_book_1
label we’ll use the StubTigger
interface as follows
stubFinder.trigger('return_book_1')
Next we’ll want to listen to the output of the message sent to jms:output
Exchange receivedMessage = camelContext.createConsumerTemplate().receive('jms:output', 5000)
And the received message would pass the following assertions
receivedMessage != null
assertThatBodyContainsBookNameFoo(receivedMessage.in.body)
receivedMessage.in.headers.get('BOOK-NAME') == 'foo'
Scenario 2 (output triggered by input)
Since the route is set for you it’s enough to just send a message to the jms:output
destination.
camelContext.createProducerTemplate().sendBodyAndHeaders('jms:input', new BookReturned('foo'), [sample: 'header'])
Next we’ll want to listen to the output of the message sent to jms:output
Exchange receivedMessage = camelContext.createConsumerTemplate().receive('jms:output', 5000)
And the received message would pass the following assertions
receivedMessage != null
assertThatBodyContainsBookNameFoo(receivedMessage.in.body)
receivedMessage.in.headers.get('BOOK-NAME') == 'foo'
Scenario 3 (input with no output)
Since the route is set for you it’s enough to just send a message to the jms:output
destination.
camelContext.createProducerTemplate().sendBodyAndHeaders('jms:delete', new BookReturned('foo'), [sample: 'header'])
Stub Runner Messaging Integration
Accurest Stub Runner’s messaging module gives you an easy way to integrate with Spring Integration. For the provided artifacts it will automatically download the stubs and register the required routes.
Adding it to the project
To use it you have to add the following dependency to your project (example for Gradle):
testCompile "io.codearte.accurest:stub-runner-messaging-integration:${accurestVersion}"
Examples
Stubs structure
Let us assume that we have the following Maven repository with a deployed stubs for the
integrationService
application.
└── .m2
└── repository
└── io
└── codearte
└── accurest
└── stubs
└── integrationService
├── 0.0.1-SNAPSHOT
│ ├── integrationService-0.0.1-SNAPSHOT.pom
│ ├── integrationService-0.0.1-SNAPSHOT-stubs.jar
│ └── maven-metadata-local.xml
└── maven-metadata-local.xml
And the stubs contain the following structure:
├── META-INF
│ └── MANIFEST.MF
└── repository
├── accurest
│ ├── bookDeleted.groovy
│ ├── bookReturned1.groovy
│ └── bookReturned2.groovy
└── mappings
Let’s consider the following contracts (let' number it with 1):
io.codearte.accurest.dsl.GroovyDsl.make {
label 'return_book_1'
input {
triggeredBy('bookReturnedTriggered()')
}
outputMessage {
sentTo('output')
body('''{ "bookName" : "foo" }''')
headers {
header('BOOK-NAME', 'foo')
}
}
}
and number 2
io.codearte.accurest.dsl.GroovyDsl.make {
label 'return_book_2'
input {
messageFrom('input')
messageBody([
bookName: 'foo'
])
messageHeaders {
header('sample', 'header')
}
}
outputMessage {
sentTo('output')
body([
bookName: 'foo'
])
headers {
header('BOOK-NAME', 'foo')
}
}
}
and the following Spring Integration Route:
<?xml version="1.0" encoding="UTF-8"?>
<beans:beans xmlns="http://www.springframework.org/schema/integration"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:beans="http://www.springframework.org/schema/beans"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/integration
http://www.springframework.org/schema/integration/spring-integration.xsd">
<!-- REQUIRED FOR TESTING -->
<bridge input-channel="output"
output-channel="outputTest"/>
<channel id="outputTest">
<queue/>
</channel>
</beans:beans>
Scenario 1 (no input message)
So as to trigger a message via the return_book_1
label we’ll use the StubTigger
interface as follows
stubFinder.trigger('return_book_1')
Next we’ll want to listen to the output of the message sent to output
AccurestMessage receivedMessage = messaging.receiveMessage('outputTest')
And the received message would pass the following assertions
receivedMessage != null
assertJsons(receivedMessage.payload)
receivedMessage.headers.get('BOOK-NAME') == 'foo'
Scenario 2 (output triggered by input)
Since the route is set for you it’s enough to just send a message to the output
destination.
messaging.send(new BookReturned('foo'), [sample: 'header'], 'input')
Next we’ll want to listen to the output of the message sent to output
AccurestMessage receivedMessage = messaging.receiveMessage('outputTest')
And the received message would pass the following assertions
receivedMessage != null
assertJsons(receivedMessage.payload)
receivedMessage.headers.get('BOOK-NAME') == 'foo'
Scenario 3 (input with no output)
Since the route is set for you it’s enough to just send a message to the input
destination.
messaging.send(new BookReturned('foo'), [sample: 'header'], 'delete')
Stub Runner Messaging Stream
Accurest Stub Runner’s messaging module gives you an easy way to integrate with Spring Stream. For the provided artifacts it will automatically download the stubs and register the required routes.
In Stub Runner’s integration with Stream the messageFrom or sentTo Strings are resolved
first as a destination of a channel, and then if there is no such destination it’s resolved as a
channel name.
|
Adding it to the project
To use it you have to add the following dependency to your project (example for Gradle):
testCompile "io.codearte.accurest:stub-runner-messaging-stream:${accurestVersion}"
Examples
Stubs structure
Let us assume that we have the following Maven repository with a deployed stubs for the
streamService
application.
└── .m2
└── repository
└── io
└── codearte
└── accurest
└── stubs
└── streamService
├── 0.0.1-SNAPSHOT
│ ├── streamService-0.0.1-SNAPSHOT.pom
│ ├── streamService-0.0.1-SNAPSHOT-stubs.jar
│ └── maven-metadata-local.xml
└── maven-metadata-local.xml
And the stubs contain the following structure:
├── META-INF
│ └── MANIFEST.MF
└── repository
├── accurest
│ ├── bookDeleted.groovy
│ ├── bookReturned1.groovy
│ └── bookReturned2.groovy
└── mappings
Let’s consider the following contracts (let' number it with 1):
io.codearte.accurest.dsl.GroovyDsl.make {
label 'return_book_1'
input {
triggeredBy('bookReturnedTriggered()')
}
outputMessage {
sentTo('returnBook')
body('''{ "bookName" : "foo" }''')
headers {
header('BOOK-NAME', 'foo')
}
}
}
and number 2
io.codearte.accurest.dsl.GroovyDsl.make {
label 'return_book_2'
input {
messageFrom('bookStorage')
messageBody([
bookName: 'foo'
])
messageHeaders {
header('sample', 'header')
}
}
outputMessage {
sentTo('returnBook')
body([
bookName: 'foo'
])
headers {
header('BOOK-NAME', 'foo')
}
}
}
and the following Spring configuration:
stubrunner.stubs.repository.root: classpath:m2repo/repository/
stubrunner.stubs.ids: io.codearte.accurest.stubs:streamService:0.0.1-SNAPSHOT:stubs
spring:
cloud:
stream:
bindings:
output:
destination: returnBook
input:
destination: bookStorage
Scenario 1 (no input message)
So as to trigger a message via the return_book_1
label we’ll use the StubTrigger
interface as follows
stubFinder.trigger('return_book_1')
Next we’ll want to listen to the output of the message sent to a channel whose destination
is returnBook
AccurestMessage receivedMessage = messaging.receiveMessage('returnBook')
And the received message would pass the following assertions
receivedMessage != null
assertJsons(receivedMessage.payload)
receivedMessage.headers.get('BOOK-NAME') == 'foo'
Scenario 2 (output triggered by input)
Since the route is set for you it’s enough to just send a message to the bookStorage
destination
.
messaging.send(new BookReturned('foo'), [sample: 'header'], 'bookStorage')
Next we’ll want to listen to the output of the message sent to returnBook
AccurestMessage receivedMessage = messaging.receiveMessage('returnBook')
And the received message would pass the following assertions
receivedMessage != null
assertJsons(receivedMessage.payload)
receivedMessage.headers.get('BOOK-NAME') == 'foo'
Scenario 3 (input with no output)
Since the route is set for you it’s enough to just send a message to the output
destination.
messaging.send(new BookReturned('foo'), [sample: 'header'], 'delete')
Migration Guide
Migration to 0.4.7
-
in 0.4.7 we’ve fixed package name (coderate to codearte) so you’ve to do the same in your projects. This means replacing
withio.coderate.accurest.dsl.GroovyDsl
io.codearte.accurest.dsl.GroovyDsl
Migration to 1.0.0-RC1
-
from 1.0.0 we’re distinguish ignored contracts from excluded contracts:
-
excludedFiles
pattern tells Accurest to skip processing those files at all -
ignoredFiles
pattern tells Accurest to generate contracts and tests, but tests will be marked as@Ignore
-
from 1.0.0 the
basePackageForTests
behaviour has changed -
prior to the change all DSL files had to be under
contractsDslDir
/basePackageForTests
/subpackage resulting inbasePackageForTests
.subpackage test package creation -
now all DSL files have to be under
contractsDslDir
/subpackage resulting inbasePackageForTests
.subpackage test package creation -
If you don’t migrate to the new approach you will have your tests under
contractsDslDir
.contractsDslDir
.subpackage
Migration to 1.1.0
-
from 1.1.0 we’re setting JUnit as a default testing utility. You have to pass the following option to keep Spock as your first choice:
targetFramework = 'Spock'